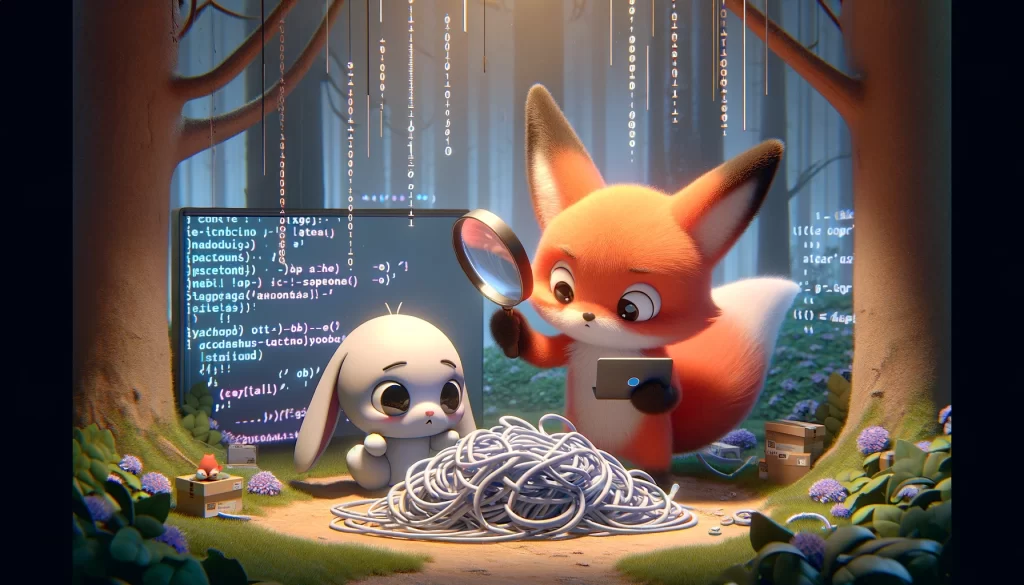
The article “Parse, Don’t Validate” by Alexis King focuses on the concept of type-driven design in programming, emphasizing the use of parsing over validation in handling data. Here’s a simplified summary:
- Type-Driven Design and Parsing: The author introduces the concept of type-driven design and suggests a shift from validating to parsing data. Parsing involves transforming less-structured input into a more-structured, type-safe format, ensuring correctness at the type level.
- Handling Partial Functions in Haskell: The article uses Haskell functions as examples to explain the concept. For instance, the
head
function, which retrieves the first element of a list, is explored. In a type-safe environment, such functions should be total (defined for all possible inputs) rather than partial (undefined for some inputs). - Transforming Partial Functions into Total Ones: Two strategies are presented for dealing with partial functions:
- Managing Expectations with Maybe: Modify the return type of a function to
Maybe
, which accounts for the possibility of not returning a valid result. For example,head
can returnNothing
for an empty list. - Strengthening Argument Types: Rather than modifying the return type, strengthen the input type to ensure only valid inputs are accepted. This is demonstrated by using a
NonEmpty
list type to ensurehead
always receives a non-empty list.
- The Power of Parsing: Parsing is preferred over validation because it preserves information about the input’s structure in the type system. Validation often discards information, while parsing retains it, leading to more robust and type-safe code.
- Avoiding Validation Pitfalls: The article discusses the dangers of ad-hoc validation, particularly the risk of “shotgun parsing,” where input validation is scattered and inconsistent, leading to potential errors and state inconsistencies.
- Practical Advice on Parsing: The author offers practical advice for implementing parsing in programming:
- Focus on data types.
- Choose data structures that make illegal states unrepresentable.
- Push for the earliest possible conversion to precise data representations.
- Refactor code iteratively to bridge gaps between ideal and current data representations.
- Reflection and Further Reading: The article concludes with reflections on the practical challenges of refactoring code for type safety and recommends further reading for those interested in exploring these concepts deeper.
In essence, the article advocates for a paradigm shift in programming towards parsing for better type safety and robustness, particularly in languages like Haskell that support strong static typing.