列出一些前端面試題目
- What is the difference between
left
in CSS andtransform
in CSS?
Answer:
The left
property and the transform
property in CSS both deal with positioning elements, but they do so in different ways and have different implications:
left
Property
- Static vs. Relative Positioning:
- The
left
property moves an element relative to its nearest positioned ancestor. - It only affects elements with a
position
value ofrelative
,absolute
,fixed
, orsticky
.
- The
- Layout Reflow:
- Using the
left
property can trigger a reflow, which means the browser recalculates the positions and geometries of elements, potentially causing performance issues for large or complex layouts.
- Using the
- No 3D Transformations:
- The
left
property only moves elements in 2D space, meaning you can move elements horizontally but cannot rotate, scale, or skew them.
- The
- Affects Layout:
- Moving an element using
left
can affect the layout of other elements, potentially causing other elements to shift or overlap.
- Moving an element using
transform
Property
- Transformation Functions:
- The
transform
property applies transformations to an element, includingtranslate
,rotate
,scale
, andskew
. - For horizontal movement similar to
left
, you would usetransform: translateX(value)
.
- The
- No Layout Reflow:
- The
transform
property does not trigger a reflow; instead, it uses the GPU for rendering, which can be more efficient and result in smoother animations.
- The
- 3D Transformations:
- The
transform
property supports both 2D and 3D transformations, allowing for more complex animations and effects.
- The
- Doesn’t Affect Layout:
- Transformations using the
transform
property do not affect the layout of other elements. The element is visually moved, but its original space in the layout is preserved.
- Transformations using the
2. variables defined in an IIFE are not in the global scope?
Yes, Variables defined inside an Immediately Invoked Function Expression (IIFE) are not in the global scope. They are scoped to the function itself.
(function() {
var bar = ” World”; // ‘bar’ is scoped to this function
})();
console.log(bar); // ReferenceError: bar is not defined
3. Why use semantic HTML?
Using semantic HTML is important for several reasons:
- Improved Accessibility: Semantic elements provide meaning to web content, making it easier for screen readers and assistive technologies to understand and navigate the page. This enhances accessibility for users with disabilities.
- Better SEO: Search engines use the semantic structure of HTML to better understand the content of web pages, which can improve search engine rankings and visibility.
- Easier Maintenance: Semantic HTML makes the code more readable and understandable, which helps developers to maintain and update the code more easily.
- Consistent Styling: By using semantic tags, you can apply consistent styling across similar elements, making it easier to manage and change the design.
- Enhanced Usability: Semantic HTML provides a clear structure, helping users to navigate the website more intuitively and understand the content better.
- Future-proofing: Using semantic HTML ensures that your code adheres to current web standards, making it more likely to be compatible with future technologies and browsers.
Incorporating semantic HTML into your web development practices leads to a more robust, accessible, and maintainable web experience. 🌐✨
What, Why & How of Semantic HTML
4. What is the same-origin policy?
The same-origin policy (SOP) is a critical security feature implemented in web browsers to prevent malicious scripts on one page from accessing data on another page unless they share the same origin. An “origin” is defined by the combination of the scheme (protocol), hostname (domain), and port of a URL. The policy helps protect against various security vulnerabilities, such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
Here’s how the same-origin policy works:
- Scheme: The protocol used (e.g., HTTP, HTTPS).
- Hostname: The domain name (e.g., www.example.com).
- Port: The port number (e.g., 80 for HTTP, 443 for HTTPS).
Two URLs are considered to have the same origin if they share the same scheme, hostname, and port. For example:
- Same Origin:
https://www.example.com/page1
andhttps://www.example.com/page2
http://www.example.com:8080
andhttp://www.example.com:8080
- Different Origin:
http://www.example.com
andhttps://www.example.com
(different scheme)https://www.example.com
andhttps://sub.example.com
(different hostname)https://www.example.com
andhttps://www.example.com:8080
(different port)
Key Points of Same-Origin Policy:
- Access Control: JavaScript can only access resources (e.g., cookies, DOM) from the same origin, preventing unauthorized access to data on different origins.
- Data Protection: It protects sensitive data by ensuring that malicious scripts cannot interact with other websites’ data.
- Exceptions: There are controlled ways to bypass the same-origin policy, such as Cross-Origin Resource Sharing (CORS), which allows servers to specify who can access their resources from different origins.
Benefits of Same-Origin Policy:
- Security: It prevents malicious activities by isolating potentially harmful scripts to their origin.
- Privacy: Protects user data by restricting unauthorized access across different origins.
- Integrity: Ensures that interactions are limited to trusted origins, maintaining the integrity of web applications.
The same-origin policy is a fundamental aspect of web security, ensuring safe and secure interactions on the web. 🔒🌐
Same-origin policy (SOP) | Web Security Academy
5. arrow function的 this
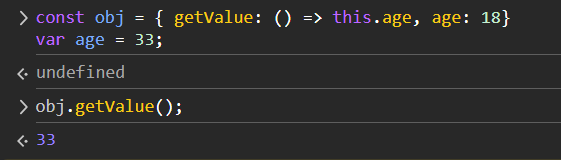
6. What are closures
閉包就好像你參加一個party,party是一個function,你知道裡面的變數的值(參加的人…),party結束了,你離開了,卻還記得party裡的東西。
一些面試題庫整理連結:
搜尋javascript interview questions就一大堆
sudheerj/javascript-interview-questions: List of 1000 JavaScript Interview Questions (github.com)
超推薦!⊙o⊙寫的簡短又好懂